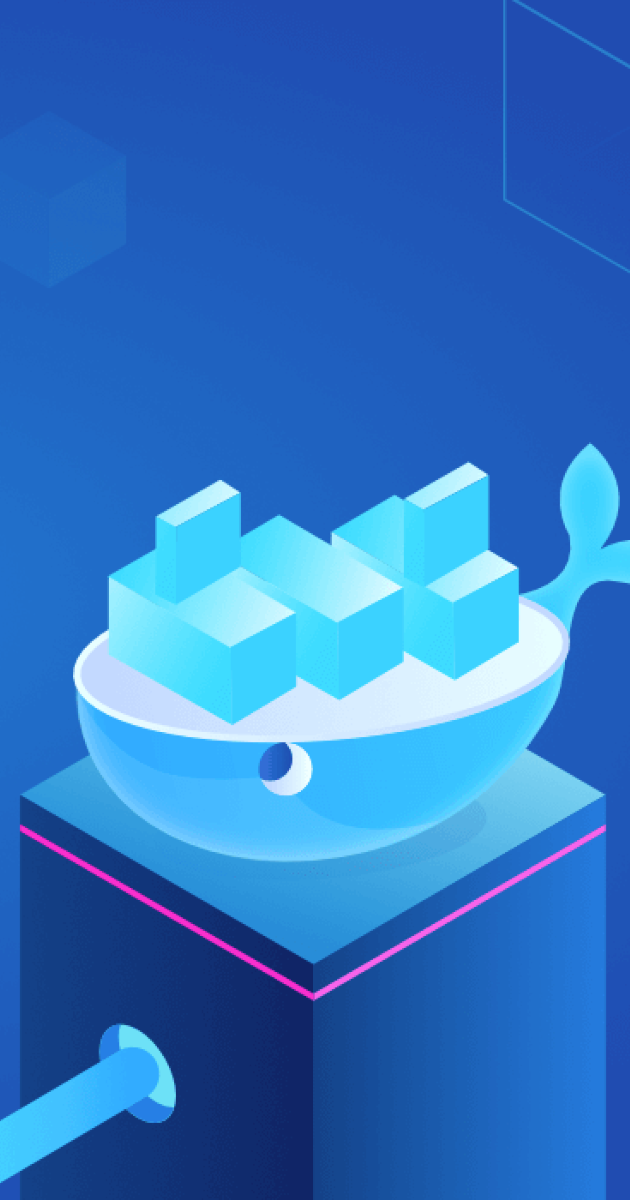
Building a Wholesale Retail System with Microservices & Event-Driven Architecture - Part 1
meocuteequas • Feb 25, 2025Welcome to this comprehensive guide on implementing a robust wholesale retail system using microservices and event-driven architecture. In this first installment, we'll explore the fundamental concepts behind this architectural approach, discuss its advantages for enterprise applications, and walk through the initial setup of our project.
Understanding Microservices Architecture
Microservices architecture represents a departure from monolithic application design by decomposing applications into smaller, specialized services that operate independently while communicating through well-defined interfaces.
Key Benefits of Microservices
The microservices approach offers several strategic advantages:
- Single Responsibility: Each service focuses on a specific business capability, enhancing maintainability
- Independent Deployment: Services can be developed, tested, and deployed individually
- Technology Diversity: Teams can select the optimal technology stack for each service
- Isolated Scalability: Resources can be allocated precisely where needed
- Resilience: System failures remain isolated to specific services rather than affecting the entire application
System Architecture Overview
Our wholesale retail system will implement a network of services that communicate through event-driven patterns. When significant state changes occur in one service, other interested services receive notifications via events. Here's the architectural composition:
- API Gateway: Serves as the entry point for all client requests, handling routing and cross-cutting concerns
- IAM Service: Manages authentication and authorization across the system
- Inventory Service: Handles product catalog and stock management
- Order Service: Processes customer orders from placement through fulfillment
- RabbitMQ: Acts as the message broker for asynchronous communication between services
- Client Portal: Provides the user interface for customer interactions
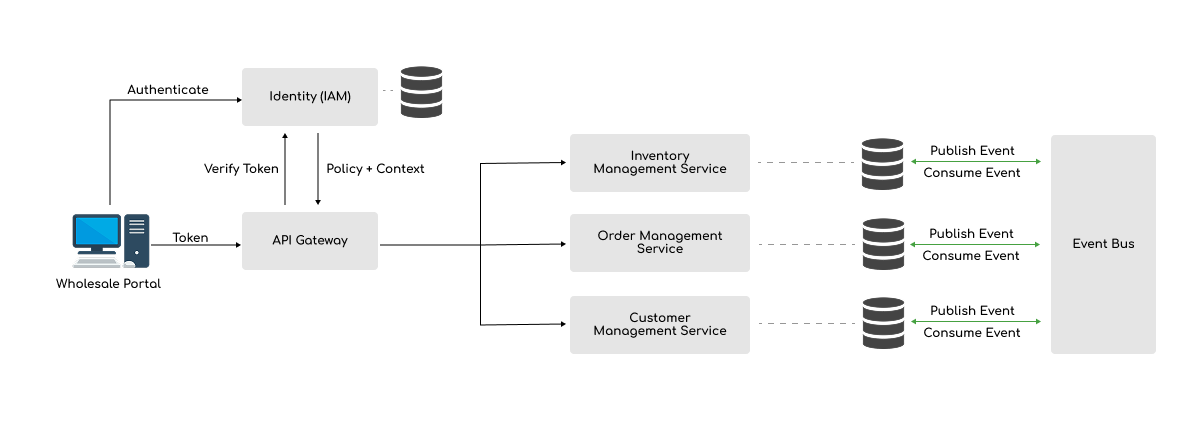
Technology Stack Selection
For this implementation, we've selected the following technologies:
- API Gateway: .NET with YARP (Yet Another Reverse Proxy)
- IAM Service: Keycloak for robust identity and access management
- Order & Inventory Services: .NET 8 Web APIs
- RabbitMQ: Enterprise-grade message broker
- Client Portal: Next.js for a responsive, modern frontend
Development Environment Setup
Before writing any code, let's establish our development environment with the following tools:
- Docker: For containerization and consistent environments
- Rider: JetBrains IDE optimized for .NET microservices development
- .NET 8: Latest framework for building high-performance backend services
- RabbitMQ: For implementing our event-driven messaging infrastructure
1. Project Structure Creation
Let's begin by setting up our solution structure in Rider:
- Create a new solution named
Wholesale System
- Add two API projects:
Gateway Service
Inventory Service
- Select
net8.0
as the target framework - Choose the Web API template
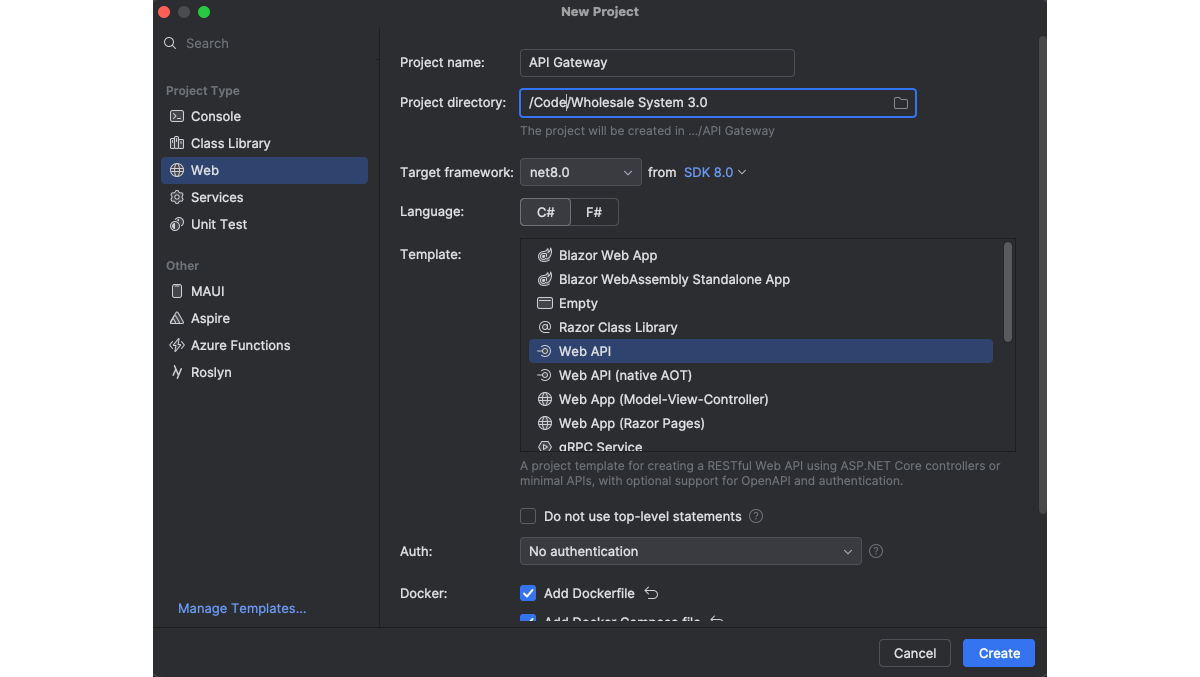
- Add Docker Compose to the solution to facilitate containerized development and debugging
When complete, your project structure should resemble the following (note: red filenames indicate files not yet added to version control):
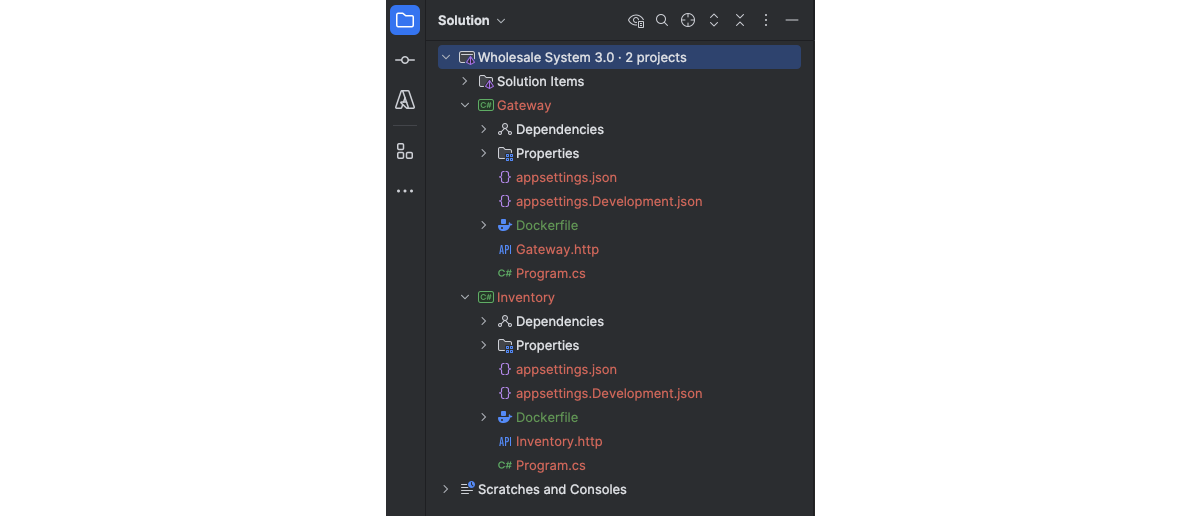
2. Docker Compose Configuration
Now that we have our project structure, we need to configure our containerized environment with the required services: Keycloak for identity management, PostgreSQL databases for persistence, and networking to enable service communication.
Open your compose.yml file and update it with the following configuration:
services: keycloak: image: quay.io/keycloak/keycloak:latest container_name: keycloak environment: KEYCLOAK_ADMIN: admin KEYCLOAK_ADMIN_PASSWORD: admin KC_DB: postgres KC_DB_URL_HOST: keycloak-db KC_DB_URL_PORT: 5432 KC_DB_USERNAME: keycloak KC_DB_PASSWORD: keycloak_password KC_DB_SCHEMA: public KC_DB_URL_DATABASE: keycloak command: start-dev ports: - "8080:8080" depends_on: - keycloak-db networks: - db-network - backend-network gateway: image: gateway container_name: gateway build: context: . dockerfile: Gateway/Dockerfile ports: - "8082:8080" - "8083:8081" networks: - backend-network inventory: image: inventory container_name: inventory build: context: . dockerfile: Inventory/Dockerfile networks: - backend-network - db-network inventory-db: image: postgres:17.4 container_name: inventory-db environment: POSTGRES_USER: inventory POSTGRES_PASSWORD: inventory_password POSTGRES_DB: inventory volumes: - inventory-data:/var/lib/postgresql/data networks: - db-network keycloak-db: image: postgres:17.4 container_name: keycloak-db environment: POSTGRES_USER: keycloak POSTGRES_PASSWORD: keycloak_password POSTGRES_DB: keycloak volumes: - keycloak-data:/var/lib/postgresql/data networks: - db-network networks: backend-network: driver: bridge db-network: driver: bridge volumes: keycloak-data: inventory-data:
This configuration establishes:
- Keycloak service on port 8080 connected to a dedicated PostgreSQL database
- Two separate networks:
db-network
for database connections andbackend-network
for inter-service communication - Volume mounts for database persistence
- Internal service communication through Docker's DNS resolution
Note that services without exposed ports are accessible only within the Docker network, with the API Gateway handling external access.
3. Implementing YARP in the Gateway Service
What is YARP?
YARP (Yet Another Reverse Proxy) is an open-source .NET library that provides robust reverse proxy capabilities. It enables:
- Centralized request routing to backend services
- Load balancing across service instances
- Request transformation and middleware integration
Configuring YARP
First, add the YARP NuGet package to your Gateway project:
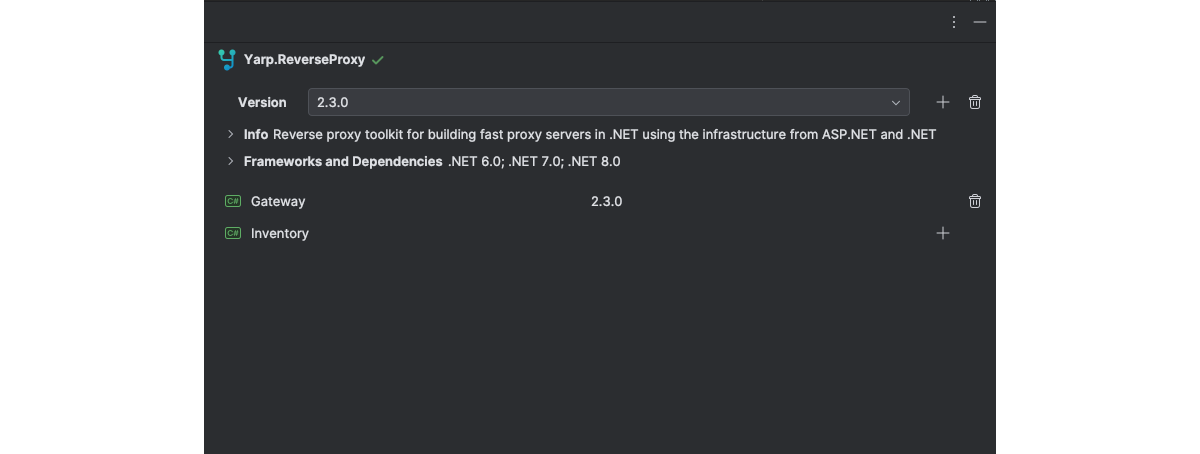
Now, update your Program.cs
file to configure YARP:
using Microsoft.AspNetCore.Authentication.JwtBearer; using Microsoft.IdentityModel.Tokens; var builder = WebApplication.CreateBuilder(args); builder.Services.AddReverseProxy() .LoadFromConfig(builder.Configuration.GetSection("ReverseProxy")); var app = builder.Build(); app.MapReverseProxy(); app.Run();
Next, configure the routing rules in appsettings.json
:
{ "Logging": { "LogLevel": { "Default": "Information", "Microsoft.AspNetCore": "Warning" } }, "AllowedHosts": "*", "ReverseProxy": { "Routes": { "inventory": { "ClusterId": "inventory", "Match": { "Path": "inventory/{**catch-all}" }, "Transforms": [ { "PathPattern": "{**catch-all}" } ] } }, "Clusters": { "inventory": { "Destinations": { "primary": { "Address": "http://inventory:8080" } } } } } }
This configuration creates a route that directs any request with the path prefix /inventory/
to the inventory service. The {**catch-all}
syntax captures all remaining path segments, which are then forwarded to the destination service.
Note: Within Docker networking, services can reference each other by container name (http://inventory:8080
), which simplifies service discovery and communication.
4. Testing the Initial Setup
With our configuration complete, let's start the application and verify that everything is working correctly:
- Run the Docker Compose configuration from your IDE
- Wait for all five containers to start: Gateway, Inventory, Keycloak, and two PostgreSQL instances
- Test the API Gateway by navigating to
http://localhost:8082/inventory/weatherforecast
in your browser
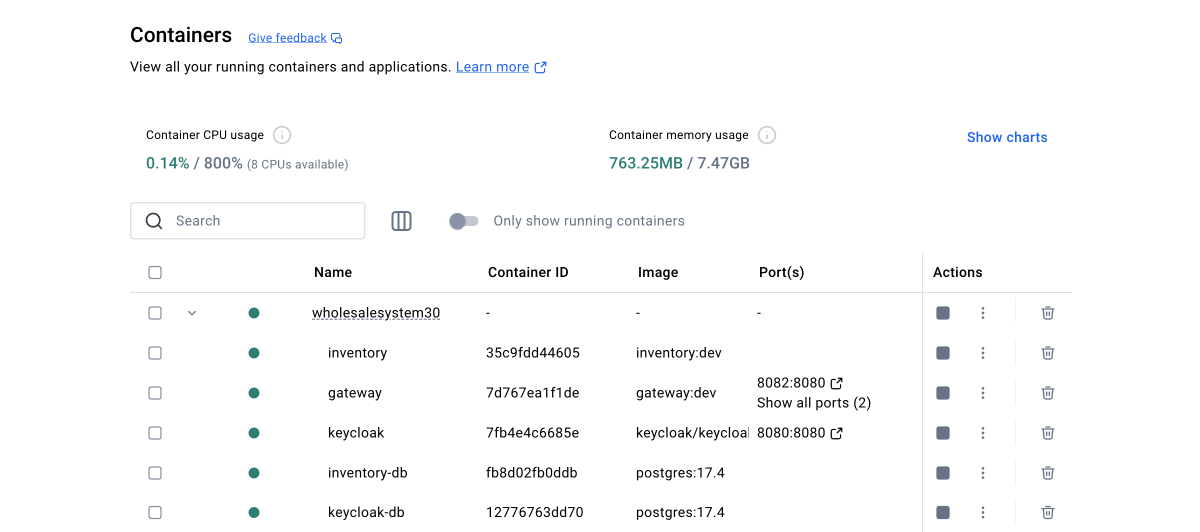
If successful, you should see the Weather Forecast JSON response from the Inventory service. Examining the Gateway service logs, you'll see entries confirming the request proxying:
info: Yarp.ReverseProxy.Forwarder.HttpForwarder[9] 2025-03-01T07:35:41.544104835Z Proxying to http://inventory:8080/weatherforecast HTTP/2 RequestVersionOrLower 2025-03-01T07:35:41.608422793Z info: Yarp.ReverseProxy.Forwarder.HttpForwarder[56] 2025-03-01T07:35:41.608455085Z Received HTTP/1.1 response 200.
This confirms that the Gateway successfully routed your request from http://localhost:8082/inventory/weatherforecast
to http://inventory:8080/weatherforecast
.
Conclusion
In this first part of our tutorial series, we've established the foundation for our wholesale retail system using microservices architecture. We've:
- Set up our project structure with .NET 8
- Configured Docker containers for our services and dependencies
- Implemented an API Gateway with YARP for request routing
- Confirmed end-to-end connectivity through our API Gateway to the Inventory service
In the next part, we'll expand our system by implementing the Inventory service functionality, adding authentication with Keycloak, and setting up event-based communication with RabbitMQ.
Stay tuned for Part 2, where we'll continue building this robust microservices ecosystem.