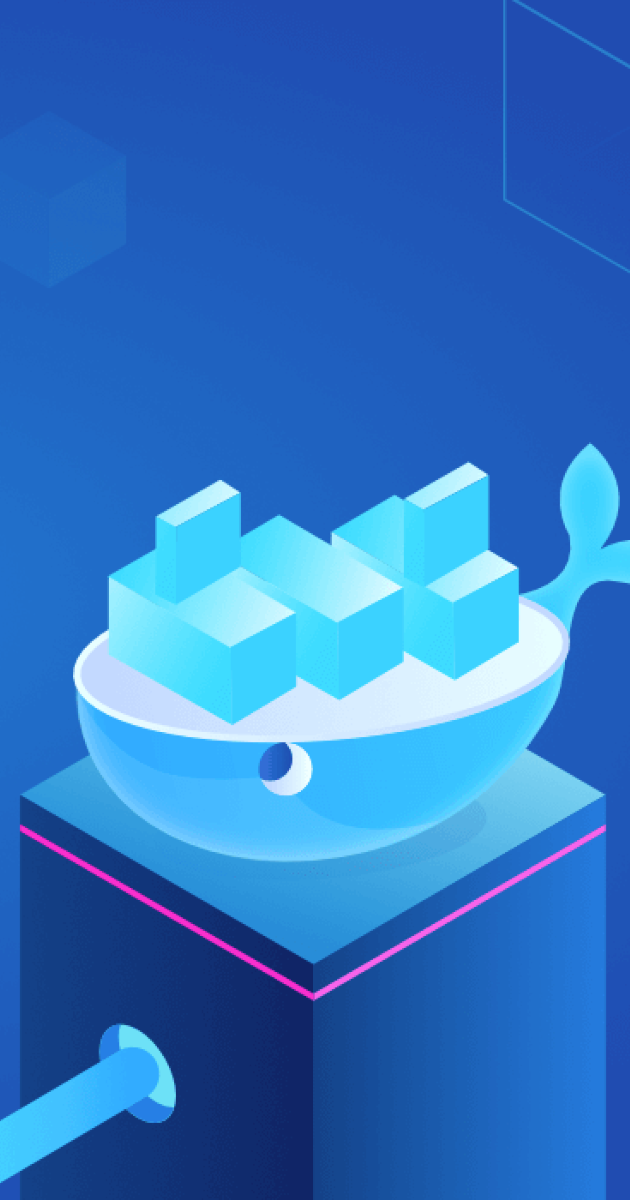
Building a Wholesale Retail System with Microservices & Event-Driven Architecture - Part 2
meocuteequas • Mar 10, 2025Welcome back to our comprehensive series on building a wholesale retail system using microservices and event-driven architecture. In part 1, we established our project foundation by setting up the development environment, configuring Docker containers, and implementing a basic API Gateway with YARP. Now, we're ready to tackle one of the most critical aspects of any distributed system: authentication and security.
The Challenge of Identity Management in Microservices
When transitioning from monolithic to microservices architecture, identity and access management becomes significantly more complex. Rather than a single application handling authentication, we now have multiple independent services that all need to verify user identity and permissions. This presents several key challenges:
- How to provide single sign-on (SSO) across all services
- How to standardize security token format and validation
- How to maintain consistent user roles and permissions
- How to avoid duplicating authentication logic across services
Fortunately, Keycloak—which we've already included in our Docker setup—offers a powerful solution to these challenges by providing a centralized identity and access management service.
Configuring Keycloak as Our Identity Provider
Accessing the Admin Console
Let's begin by ensuring our Keycloak instance is running properly and configuring it for our wholesale system needs.
- Open your browser and navigate to
http://localhost:8080
- You'll see the Keycloak welcome page with a link to the "Administration Console"
- Click on this link and log in with the credentials we defined in our Docker Compose file:
- Username:
admin
- Password:
admin
- Username:
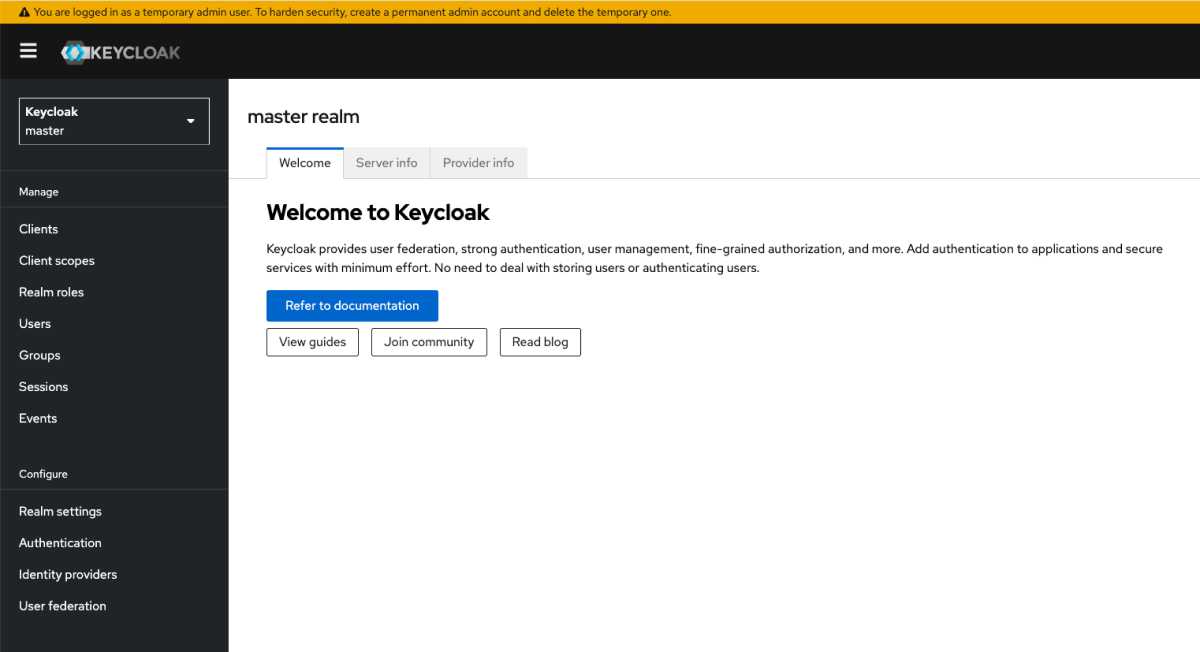
Creating a Dedicated Realm
In Keycloak terminology, a "realm" represents a security boundary containing users, applications, roles, and groups. Rather than using the default "Master" realm (which is intended for Keycloak's own administration), we'll create a custom realm for our wholesale system.
- Hover over "Master" in the upper-left corner and click "Create Realm"
- Enter "meocuteequas" as the realm name
- Click "Create" to establish our new realm
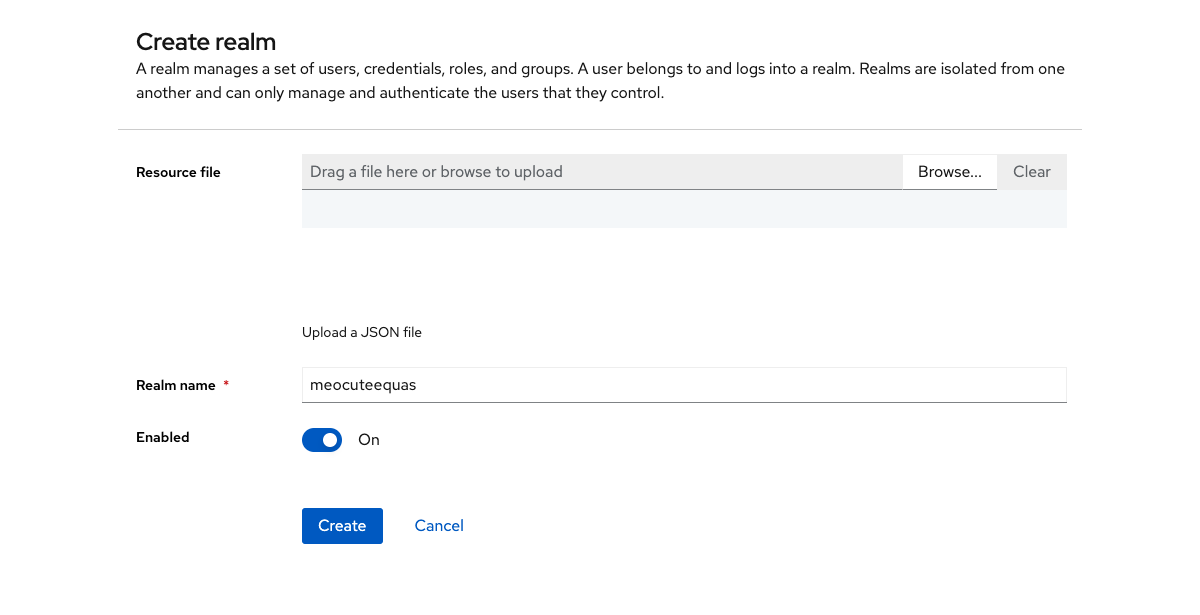
This creates an isolated security context specifically for our wholesale application ecosystem.
Registering Our Client Application
Next, we need to register our application as a "client" in Keycloak. This establishes the trust relationship between Keycloak and our system.
-
Navigate to "Clients" in the left sidebar
-
Click "Create client"
-
Configure the basic settings:
- Client ID:
meocuteequas-confidential-client
(this identifies our application to Keycloak) - Client Authentication:
ON
(this makes it a confidential client that uses a secret) - Click "Next"
- Client ID:
-
Configure the authentication flow settings:
- Valid redirect URIs:
http://localhost:3000/api/auth/callback/keycloak
(where users will be redirected after authentication) - Valid post logout redirect URIs:
http://localhost:3000
(where users will be redirected after logging out) - Web origins:
http://localhost:3000
(allowed origins for CORS) - Click "Save"
- Valid redirect URIs:
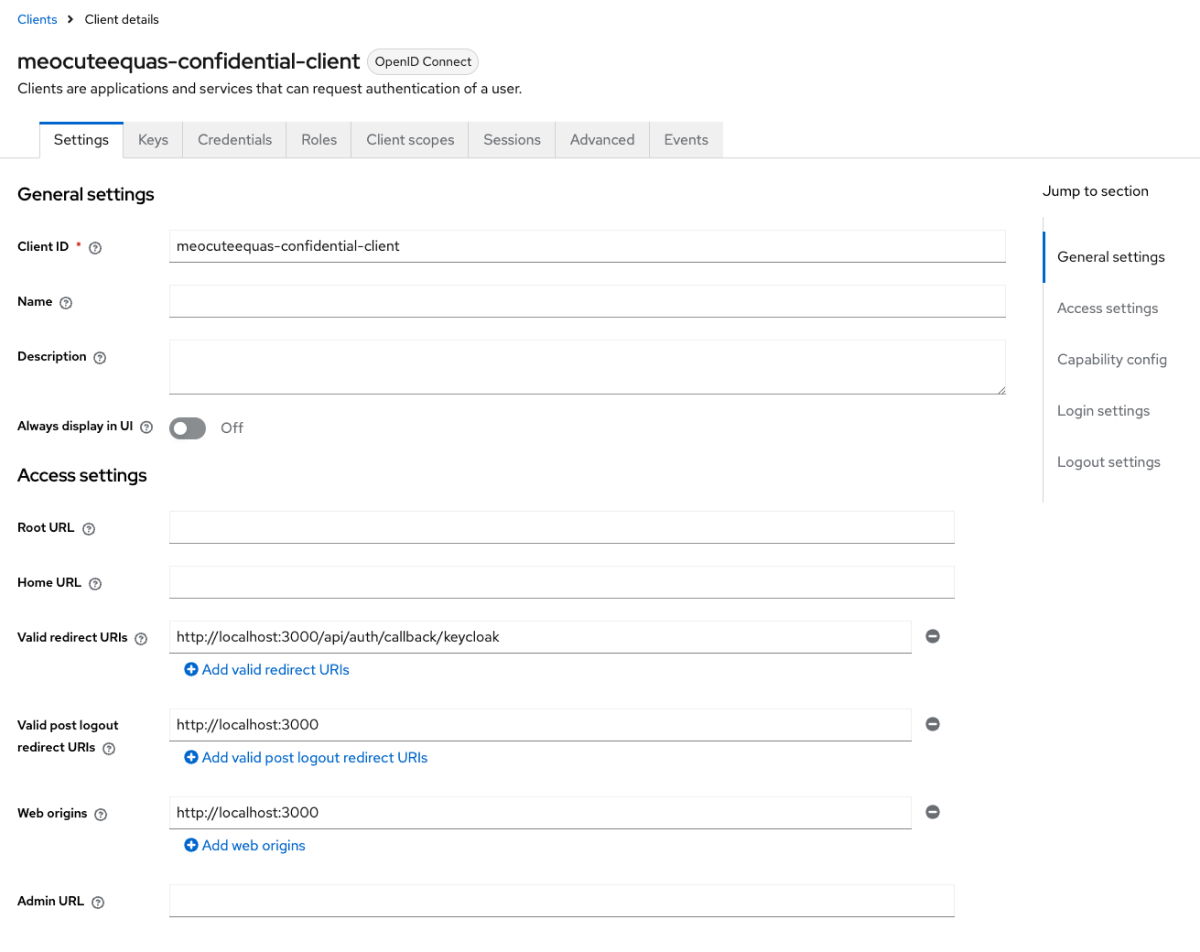
Setting Up Client Scopes and API Access
To define what our client can access, we'll create a dedicated client scope:
- Navigate to "Client scopes" in the left sidebar
- Click "Create client scope"
- Name it
wholesale-api
- Set type to "Optional"
- Click "Save"
Now we need to assign this scope to our client:
- Return to your newly created client
- Select the "Client scopes" tab
- Click "Add client scope"
- Select the "wholesale-api" scope
- Click "Add"
This establishes what resources our application is permitted to access.
Creating Test Users
For development and testing purposes, let's create a sample user:
-
Navigate to "Users" in the left sidebar
-
Click "Add user"
-
Complete the user profile:
- Username:
admin
- First Name:
Tom
- Last Name:
Admin
- Email:
[email protected]
- Email Verified:
ON
(to bypass verification steps) - Click "Create"
- Username:
-
After creation, configure credentials:
- Go to the "Credentials" tab
- Click "Set password"
- Enter password:
password
- Temporary:
OFF
(so the user doesn't need to change it on first login) - Click "Save"
With these configurations in place, our Keycloak instance is now ready to handle authentication for our wholesale system.
Securing the API Gateway with JWT Authentication
Now that our identity provider is configured, let's update our API Gateway to validate JWT tokens issued by Keycloak, ensuring that only authenticated users can access our services.
Adding Required Authentication Packages
First, we need to add the JWT Bearer authentication package to our Gateway service:
Microsoft.AspNetCore.Authentication.JwtBearer
This package provides middleware for validating JWT tokens according to the OAuth 2.0 and OpenID Connect standards.
Implementing JWT Authentication in the Gateway
Now, let's modify the Gateway's Program.cs
file to incorporate JWT authentication:
var builder = WebApplication.CreateBuilder(args); // Add JWT authentication builder.Services.AddAuthentication(JwtBearerDefaults.AuthenticationScheme) .AddJwtBearer(options => { options.RequireHttpsMetadata = false; options.Audience = "meocuteequas-confidential-client"; options.MetadataAddress = "http://keycloak:8080/realms/meocuteequas/.well-known/openid-configuration"; options.TokenValidationParameters = new TokenValidationParameters { ValidIssuer = "http://localhost:8080/realms/meocuteequas", }; }); builder.Services.AddAuthorization(); // Add YARP reverse proxy builder.Services.AddReverseProxy() .LoadFromConfig(builder.Configuration.GetSection("ReverseProxy")); var app = builder.Build(); // Enable authentication & authorization app.UseAuthentication(); app.UseAuthorization(); app.MapReverseProxy(); app.Run();
This configuration:
- Registers the JWT Bearer authentication scheme
- Configures it to validate tokens against our Keycloak instance
- Specifies our client ID as the audience
- Points to Keycloak's OpenID Connect discovery endpoint for metadata
- Validates the token issuer
- Enables authentication and authorization middleware in the request pipeline
Applying Authorization to Routes
With authentication in place, let's update the Gateway's route configuration in appsettings.json
to require authorization:
{ "Logging": { "LogLevel": { "Default": "Information", "Microsoft.AspNetCore": "Warning" } }, "AllowedHosts": "*", "ReverseProxy": { "Routes": { "inventory": { "ClusterId": "inventory", "AuthorizationPolicy": "Default", "Match": { "Path": "inventory/{**catch-all}" }, "Transforms": [ { "PathPattern": "{**catch-all}" } ] } }, "Clusters": { "inventory": { "Destinations": { "primary": { "Address": "http://inventory:8080" } } } } } }
By adding the "AuthorizationPolicy": "Default"
property to our route, we ensure that only authenticated requests can access the inventory service through our gateway.
Testing the Authentication Flow
Let's verify our authentication setup by obtaining a token from Keycloak and using it to access our protected API.
Obtaining an Access Token
We can use the Password Grant flow to obtain an access token (note that in production, you'd typically use Authorization Code flow for web applications):
curl -X POST \ http://localhost:8080/realms/meocuteequas/protocol/openid-connect/token \ -H 'Content-Type: application/x-www-form-urlencoded' \ -d 'grant_type=password&client_id=meocuteequas-confidential-client&client_secret=YOUR_CLIENT_SECRET&username=administrator&password=password'
Replace YOUR_CLIENT_SECRET
with your client's secret, which you can find in the Keycloak admin console under your client's "Credentials" tab.
A successful response will look something like this:
{ "access_token": "eyJhbGciOiJSUzI1NiIsInR5cCIgOiAiSldUIiwia2lkIiA6ICJhYmNkZWYxMjM0NTY3ODkwMTIzNDU2Nzg5MGFiY2RlZjEyMzQ1Njc4In0...", "expires_in": 300, "refresh_expires_in": 1800, "refresh_token": "eyJhbGciOiJSUzI1NiIsInR5cCIgOiAiSldUIiwia2lkIiA6ICJhYmNkZWYxMjM0NTY3ODkwMTIzNDU2Nzg5MGFiY2RlZjEyMzQ1Njc4In0...", "token_type": "Bearer", "not-before-policy": 0, "session_state": "a1b2c3d4-e5f6-7890-a1b2-c3d4e5f67890", "scope": "email profile" }
Making an Authenticated Request
Now, let's use this token to access our protected inventory endpoint:
curl -X GET \ http://localhost:8082/inventory/weatherforecast \ -H 'Authorization: Bearer eyJhbGciOiJSUzI1NiIsInR5cCIgOiAiSldUIiwia2lkIiA6ICJhYmNkZWYxMjM0NTY3ODkwMTIzNDU2Nzg5MGFiY2RlZjEyMzQ1Njc4In0...'
If everything is configured correctly, you'll receive the WeatherForecast JSON response. Without a valid token, you'd get a 401 Unauthorized response instead.
Developing a Next.js Client Portal
Now that our backend authentication is set up, let's create a user-friendly client portal using Next.js and Auth.js to interact with our services.
Creating the Next.js Application
Let's start by creating a new Next.js application. If you need detailed instructions, check out the Next.js documentation for a step-by-step guide.
Adding Authentication with Auth.js
Next, we'll integrate Auth.js (formerly NextAuth.js) to handle authentication in our client application. Follow the Auth.js documentation for detailed installation instructions.
Configuring Keycloak Authentication
Once Auth.js is installed, we need to configure it to work with our Keycloak instance:
- Create a
.env.local
file in your Next.js project with the following content:
AUTH_SECRET=YOUR_AUTH_SECRET # Added by `npx auth`. Read more: https://cli.authjs.dev AUTH_KEYCLOAK_ID=meocuteequas-confidential-client AUTH_KEYCLOAK_SECRET=YOUR_KEYCLOAK_SECRET
- Create an
auth.js
file to configure Auth.js with our Keycloak provider:
import NextAuth from "next-auth"; import Keycloak from "next-auth/providers/keycloak"; export const { handlers, signIn, signOut, auth } = NextAuth({ providers: [Keycloak], callbacks: { async jwt({ token, account }) { if (account) { token.accessToken = account.access_token; } return token; }, async session({ session, token }) { if (token) { session.accessToken = token.accessToken; } return session; }, }, session: { strategy: "jwt" }, });
This configuration:
- Sets up Keycloak as our authentication provider
- Configures callbacks to store the access token in the session
- Uses JWT strategy for session management
Creating an Auth Provider Component
To make authentication state available throughout our application, let's create an AuthProvider
context:
"use client"; import { SessionProvider } from "next-auth/react"; export default function AuthProvider({ children }: { children: React.ReactNode }) { return <SessionProvider>{children}</SessionProvider>; }
Then, update your root layout to use this provider:
<html lang="en"> <body className={`${geistSans.variable} ${geistMono.variable} antialiased`}> <AuthProvider>{children}</AuthProvider> </body> </html>
Implementing Authentication Middleware
Let's create a middleware to protect our routes and redirect unauthenticated users to the sign-in page:
import { auth } from "./auth"; import { NextResponse } from "next/server"; import type { NextRequest } from "next/server"; export async function middleware(request: NextRequest) { const session = await auth(); if (!session) { return NextResponse.redirect(new URL("/auth/sign-in", request.url)); } return NextResponse.next(); } export const config = { matcher: ["/((?!api|_next/static|_next/image|favicon.ico|auth/sign-in).*)"], };
This middleware:
- Checks if the user has an active session
- Redirects unauthenticated users to the sign-in page
- Excludes static assets and the sign-in page itself from authentication checks
Creating a Sign-In Page
Now, let's create a sign-in page at /auth/sign-in/page.tsx
:
"use client"; import { Loader2 } from "lucide-react"; import { signIn, useSession } from "next-auth/react"; import { useRouter } from "next/navigation"; import { useEffect } from "react"; export default function SignIn() { const router = useRouter(); const session = useSession(); useEffect(() => { if (session.status === "unauthenticated") { signIn("keycloak"); } if (session.status === "authenticated") { return router.push("/"); } }, [session.status, router]); useEffect(() => { document.body.classList.add("overflow-hidden"); return () => { document.body.classList.remove("overflow-hidden"); }; }, []); return ( <div className="absolute inset-0 flex items-center justify-center bg-background/80 backdrop-blur-sm z-[30]"> <div className="flex items-center"> <Loader2 className="animate-spin" size={20} /> </div> </div> ); }
This page:
- Automatically initiates Keycloak sign-in for unauthenticated users
- Redirects authenticated users back to the homepage
- Shows a loading spinner during the authentication process
Building the Homepage with Authenticated API Access
Finally, let's update our homepage to use the authenticated session to make API requests:
import { auth } from "@/auth"; export default async function Home() { const session = await auth(); const api = await fetch("http://localhost:8082/inventory/weatherforecast", { headers: { Authorization: `Bearer ${session!.accessToken}`, }, }); const data = await api.json(); return ( <div className="w-screen h-screen flex justify-center items-center flex-col"> <h1 className="text-4xl font-bold mb-4">Welcome {session!.user.name}!</h1> <p>Weather forecast data: {JSON.stringify(data)}</p> </div> ); }
Testing the Complete Authentication Flow
With everything in place, let's test our end-to-end authentication flow:
- When you first access the client portal, you'll be automatically redirected to the Keycloak login page
- After successful authentication, you'll be returned to the homepage
- The homepage will display your name from the session and fetch data from the inventory service using your access token
- The API Gateway will validate your token before forwarding the request to the Inventory service
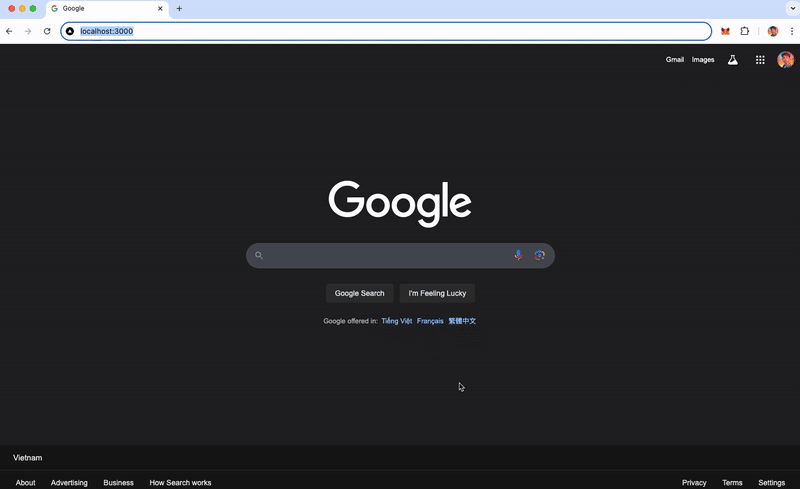
This confirms that we've successfully set up a complete authentication flow from the client through our API Gateway to our backend services.
Conclusion
In this second part of our series, we've established a comprehensive authentication infrastructure for our wholesale retail system. We've:
- Configured Keycloak as our centralized identity provider
- Set up realms, clients, and test users
- Implemented JWT authentication in our API Gateway
- Protected our routes with authorization policies
- Created a Next.js client application with seamless authentication
- Demonstrated end-to-end authenticated API access
This authentication foundation is critical for our microservices architecture, providing consistent security across all services while maintaining separation of concerns. By centralizing authentication in Keycloak, we've avoided duplicating security logic across services and created a scalable solution that can grow with our system.
In Part 3 of this series, we'll dive deeper into the business functionality of our wholesale system by implementing the core features of our Inventory service. We'll model product catalogs, manage inventory levels, and introduce event-driven communication patterns with RabbitMQ to handle updates across services.
Stay tuned as we continue building our robust, scalable wholesale retail system with microservices and event-driven architecture!