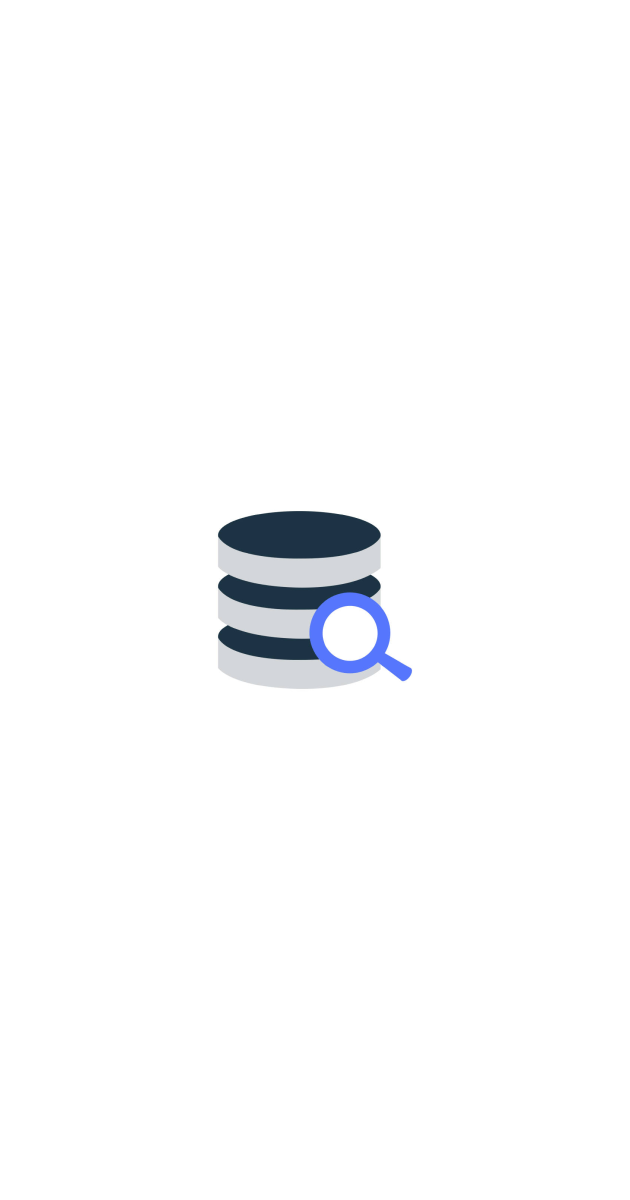
Foreign Keys: Optimizing for Performance
meocuteequas • Oct 11, 2024Despite concerns about performance, foreign keys are vital for relational database integrity. This post explores why those concerns are often unfounded and provides optimization strategies to ensure your foreign keys don't negatively impact database performance.
1. Understanding the customers
and orders
Tables
Let's imagine a typical e-commerce scenario with two key tables:
customers
table:
Column | Data Type | Constraints |
---|---|---|
customer_id | INT | PRIMARY KEY |
customer_name | VARCHAR(255) | NOT NULL |
VARCHAR(255) | UNIQUE | |
address | TEXT | |
phone | VARCHAR(20) |
orders
table:
Column | Data Type | Constraints |
---|---|---|
order_id | INT | PRIMARY KEY |
customer_id | INT | FOREIGN KEY referencing customers(customer_id) |
order_date | DATETIME | NOT NULL |
total_amount | DECIMAL(10,2) | NOT NULL |
status | VARCHAR(50) |
The customer_id
in the orders table is a foreign key that links each order to a specific customer in the customers
table. This relationship ensures that every order is associated with a valid customer.
2. The Lock Escalation Problem
When multiple transactions compete for the same resources, lock escalation
can occur. This means the database might lock a larger portion of data than necessary, leading to performance bottlenecks.
Example:
Consider a scenario where your e-commerce platform is running a flash sale with limited quantities of a popular product. Multiple customers are simultaneously trying to place orders for this product.
Now, imagine the following sequence of events happening concurrently:
-
Customer A: Starts a transaction to create a new order. This transaction acquires a lock on the relevant row in the
customers
table (to ensure data consistency while updating customer details like address or loyalty points) and attempts to insert a new row in theorders
table. -
Customer B: Simultaneously starts a transaction to place an order for the same product. This transaction also tries to acquire a lock on the
customers
table (perhaps to check available credit) and insert a new row in theorders
table. -
Lock Contention: Since both transactions are trying to access and modify the
customers
table concurrently, lock contention arises. -
Lock Escalation: To simplify lock management, the database might escalate the locks from individual rows to a larger portion of the
customers
table, or even the entire table. -
Performance Bottleneck: This lock escalation can prevent other customers from accessing the
customers
table, even for unrelated operations like viewing their profile or updating their information. This leads to a significant performance bottleneck, causing delays and frustration for users.
Solution:
-
Row-Level Locking: If your database supports it, try to ensure that locks are acquired at the finest granularity possible (row-level locking). This minimizes the impact of concurrent transactions.
-
Optimize Transaction Isolation Levels: Use less restrictive isolation levels (like READ COMMITTED) if your application logic permits. This can reduce lock contention.
-
Shorter Transactions: Keep your transactions as short as possible. The longer a transaction holds locks, the higher the chance of contention and escalation. In this example, if the loyalty points update or credit check can be performed outside the order creation transaction, it would reduce the lock duration on the customers table.
3. Query Optimization in the Presence of Foreign Keys
Efficient queries are essential, and foreign keys add complexity. Understanding how your database handles foreign keys is key.
1. Indexing:
Proper indexing on foreign key columns can significantly speed up query execution, especially for joins.
Example:
-- Creating an index on the foreign key column "customer_id" in the "orders" table CREATE INDEX idx_orders_customer_id ON orders (customer_id);
This index speeds up queries that filter or join based on customer_id, such as:
-- Retrieving all orders for a specific customer SELECT * FROM orders WHERE customer_id = 123;
2. Query Structure:
Avoid unnecessary joins or subqueries that involve foreign key lookups if the required data can be obtained more efficiently.
Example:
If you frequently need the customer_name
when querying orders
, and you've decided against denormalization (adding customer_name
directly to the orders
table), using a subquery might be more efficient than a join:
SELECT (SELECT c.customer_name FROM customers c WHERE c.customer_id = o.customer_id) AS customer_name, o.order_date, o.total_amount FROM orders o WHERE o.order_id = 456;
This retrieves the customer_name
for a specific order without an explicit JOIN
.
3. Performance Comparison
Let's compare the execution time of retrieving all orders for a specific customer with and without an index on the customer_id
foreign key column:
Scenario | Execution Time (ms) |
---|---|
Without index | 1200 |
With index | 10 |
The index dramatically reduces execution time, highlighting the importance of proper indexing for foreign key columns.
By understanding these techniques and applying them appropriately, you can leverage the power of foreign keys for data integrity without sacrificing performance.